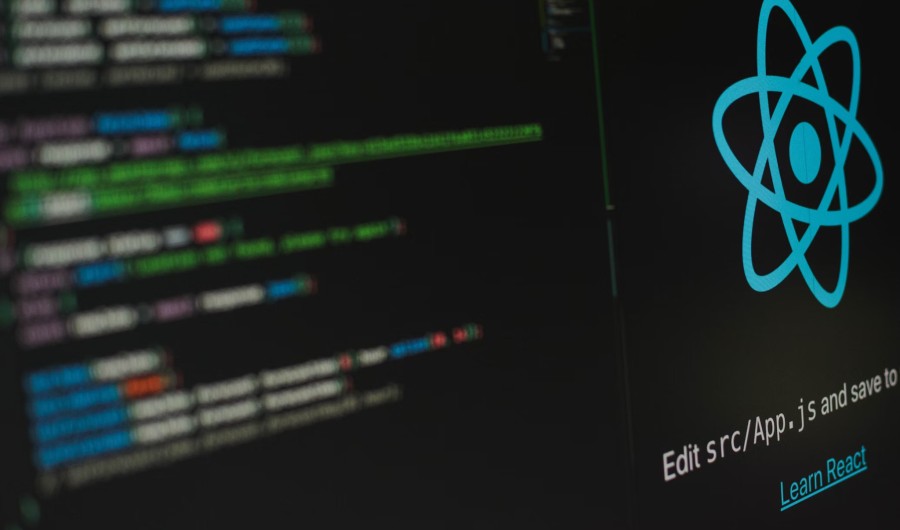
Troubleshooting React: Effective Strategies for Addressing Bugs in Your React-based Product
As a developer using React, you have likely faced that frustrating moment when your application suddenly stops working, leaving you puzzled. Rest assured, you are not alone! Although React is a powerful tool, its energetic environment can sometimes result in unexpected bugs, including rendering problems and errors related to asynchronous processes. But don’t worry! This article will explore effective strategies to help you quickly diagnose and fix bugs in your React projects. So, let’s get to work and prepare to eliminate some bugs!
Common React Errors and How to Identify Them
Before we look into the details of troubleshooting, let’s review some of the most frequent errors you may encounter on your React journey. Believe me, being aware of these will save you numerous hours of frustration!
First up, we have the infamous “too many re-renders” error. This lousy boy usually pops up when you accidentally create an infinite loop in your component’s render cycle. It’s like your component is stuck in a never-ending conversation with itself!
Next, let’s discuss AJAX/HTTP errors. These pesky issues often arise when you attempt to retrieve data from an API, but something needs improvement. It could be that the server is offline, or you may have made an error in your URL. No matter the reason, these errors can leave your application unresponsive and frustrate your users.
Lastly, we must consider component rendering failures. These issues can occur for various reasons, such as passing incorrect props to a component or forgetting to return JSX in your render method. It’s similar to attempting to assemble a puzzle with pieces that do not fit together.
So, how can you identify these errors? Your browser’s developer tools will be beneficial in this regard. The console typically provides detailed error messages that can guide you toward a solution. Also, resources like React Developer Tools can be extremely useful for issues specific to React. We will explore these tools in more detail in the upcoming section, so please stay tuned!
Debugging Tools in React
Now that we’ve identified some common errors let’s talk about the tools that’ll help you track them down and squash them like your coding pro!
First up, we have the React Developer Tools. This browser extension is a universal tool for React debugging. It lets you inspect your component hierarchy, check out the props and state of your components, and even see how your app is re-rendering. It’s beneficial for tracking down those pesky ReactJS performance issues.
Next, we have the debugging features in VS Code. If you’re not using these, you’re missing out! With breakpoints and step-through debugging, you can pause your code execution and examine variables at any point. It’s like having X-ray vision for your code!
And let’s not forget our old friend, console logging. While it might seem basic, strategically placed console.log() statements can be conducive for tracking data flow through your app and spotting where things go wrong.
Error Boundaries and Their Role
Now, let’s discuss a React-specific feature that can be a game-changer when it comes to handling errors: Error Boundaries.
Error boundaries are like the safety nets of the React world. They’re particular components that catch JavaScript errors anywhere in their child component tree, log those errors, and display a fallback UI instead of the component tree that crashed. In other words, they prevent your entire app from going down in flames just because one component decided to throw a tantrum.
But here’s the thing: error boundaries differ from regular try-catch blocks. While try-catch is great for imperative code, error boundaries are explicitly designed for declarative React components. They can catch errors during rendering, in lifecycle methods, and constructors of the whole tree below them.
To implement an error boundary, you must define a class component that implements the componentDidCatch() lifecycle method. This method acts as a catch block for components. Any error thrown in a child component will be passed to this method, allowing you to handle it gracefully.
Handling Asynchronous Bugs (AJAX/HTTP Errors)
Let’s tackle one of the trickiest areas of React development: handling asynchronous operations. It is crucial to deal with async bugs when optimizing React application performance efficiently.
Async bugs often rear their ugly heads when you’re making API calls or working with promises. Maybe your API call failed, or perhaps you forgot to handle a rejected promise. These issues can leave your app in a loading state forever or crash it entirely.
So, how do we handle these slippery customers? First and foremost, always use proper error handling.
If you’re using async/await (and you probably should be; it’s fantastic!), remember to wrap your async operations in try-catch blocks.
Another pro tip: implement retry strategies for your API calls. Sometimes, a request might fail due to a temporary network glitch. By automatically retrying the request a few times, you can recover from these transient errors without the user noticing.
Performance Bottlenecks and Rendering Issues
Now, let us shift our focus to performance. After all, what is the benefit of having a bug-free application if it operates slower than a snail on vacation? In terms of React performance optimization, recognizing and resolving rendering issues is essential.
Unnecessary re-rendering is a frequent performance bottleneck in React applications. This occurs when a component re-renders even when its props or state remains unchanged. It’s like repainting your entire house every time you replace a lightbulb!
The React Profiler is an excellent tool for determining these issues. It is included in the React Developer Tools and enables you to record a session of your application to see which components are rendering and how long they take. It’s like having a stopwatch for each component!
Once you’ve identified problematic components, there are several strategies you can use for React performance optimization:
- Memoization: Use React.memo() for functional components or PureComponent for class components to prevent unnecessary re-renders.
- Splitting components: Break large components into smaller, more focused ones. This can help React update only the UI parts that need to change.
- Use the useCallback and useMemo hooks: These hooks can help you optimize the performance of your functional components by memoizing functions and computed values.
Remember, the key to optimizing React app performance is to measure first, then optimize. Don’t fall into the trap of premature optimization!
Best Practices for Preventing Bugs
As the saying goes, an ounce of prevention is worth a pound of cure. The same is true when it comes to React bugs. By following some best practices, you can catch many issues before they even make it to production.
Here are some key preventative measures:
- Write tests (unit, integration)
- Use PropTypes or TypeScript
- Employ error boundaries
- Keep dependencies updated
Writing tests is like having a safety net for your code. Unit tests can help you catch issues in individual components or functions, while integration tests ensure that different parts of your app work well together.
Using PropTypes or TypeScript adds an extra layer of type safety to your code. It’s like having a spell-checker for your props and state! This can catch many bugs at compile time before they can cause issues at runtime.
We’ve already discussed error boundaries, but they’re worth mentioning again. By strategically placing error boundaries in your app, you can prevent entire sections from crashing due to a bug in a single component.
Lastly, keeping your dependencies up-to-date is crucial. New versions often include bug fixes and performance improvements. Test thoroughly after updating, as major versions can sometimes introduce breaking changes.
Conclusion
Wow! In our exploration of troubleshooting React performance optimization, we have covered an important amount of information. You now possess a set of strategies to address common errors, use debugging tools, manage asynchronous bugs, and enhance performance, equipping you to handle any React issue that arises.
Keep in mind that effective troubleshooting involves both prevention and resolution. You can develop more resilient and high-performing React applications by adhering to best practices, employing appropriate tools, and systematically addressing issues.
So, when you face a bug in your React application, remain calm! Take a moment to breathe deeply, gather your debugging tools, and tackle the challenge step by step. With experience, you will be debugging like an expert in no time. Happy coding!