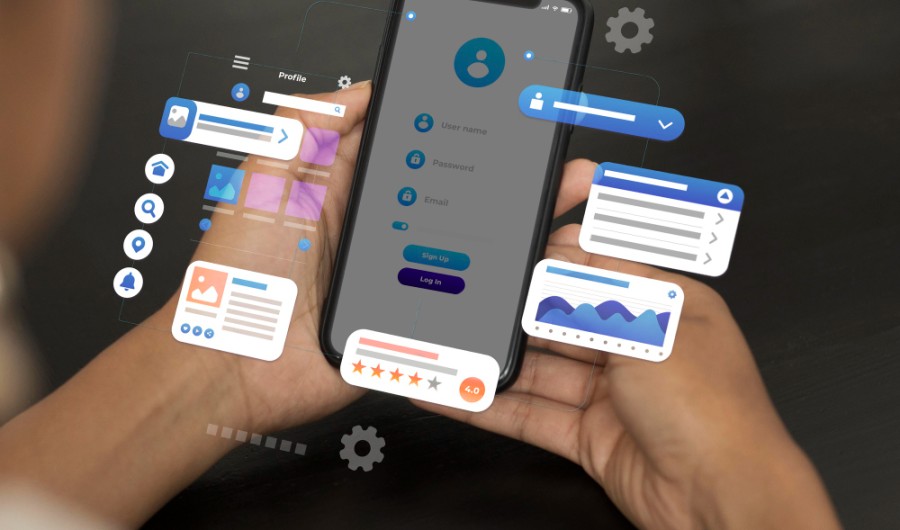
Boosting Your Android Apps With Kotlin: A Developer’s Guide
Kotlin has grown into a go-to language for Android app development, offering developers enhanced productivity and better apps. Let’s look at some numbers – according to data from 2023, more than 60% of professional Android developers use Kotlin as their primary language. On top of that 95% of the top 1,000 apps on the Google Play Store are built using Kotlin.
Photo by Desola Lanre-Ologun on Unsplash
Things are really going well for Kotling since Google’s endorsed it in with integration with Android Studio and Jetpack libraries. This skyrocketed its adoption across the Android ecosystem.
Kotlin has built-in features going for it, such as null safety and coroutines, help reduce app crashes by up to 20% and improve asynchronous programming. Kotlin’s concise syntax allows developers to write code faster and with fewer errors compared to Java.
This shift towards Kotlin reflects its ability to streamline the development process, making it a top choice for modern Android apps.
Kotlin Functions For Android Developers
Kotlin offers far too many powerful features to cover all in this piece so we’ve decied to give a couple of areas a more detailed look.
One of the key areas where Kotlin shines is in its handling of functions. Kotlin’s expressive syntax allows developers to write concise and flexible functions that cater to various Android app requirements.
We’ll explore key Kotlin functions, including handling dates and times, higher-order functions, extension functions, and lambda expressions—each essential for Android developers.
Working With Dates And Times In Kotlin
Handling dates and times is a fundamental task in most Android applications. This is used for all the commonplace tasks – scheduling notifications, displaying timestamps and setting reminders. Kotlin provides several options for working with dates and times.
The meat and potatoes way to manage dates and times in Kotlin is using java.time, which was introduced with Java 8. This package provides run of the mill classes like LocalDate, LocalTime, and LocalDateTime, which are widely used for date and time operations. For example, you can get the most basic function to get current date and time using LocalDateTime.now() and format it using DateTimeFormatter:
For Android developers handling time zones, java.time.ZonedDateTime provides a way to manage and convert between different time zones. This is especially useful in applications that serve global users. Here’s how you can manage time zones:
Kotlin also has a multiplatform library, kotlinx-datetime, which provides a simpler API for working with dates and times across different platforms. The library can be particularly useful for scheduling tasks or managing recurring events in your application.
Utilizing Higher-Order Functions
Higher-order functions make the language more expressive and flexible. A higher-order function is basically a function that is able to take another function as a parameter or returns a function. This opens the doors for developers to write reusable code (which is a big timesaver) that can be easily changed to fit many functions.
For example, Android developers often need to perform network calls asynchronously. Kotlin coroutines, combined with higher-order functions, simplify asynchronous programming:
In this example, callback is a higher-order function that takes a string as input and allows the caller to define what should be done with the network result. This basically means that you get at least two steps for the price of one.
Higher-order functions are also common in event handling, where you can pass functions that define specific behaviors, such as click listeners in Android’s UI components.
Leveraging Extension Functions
Kotlin’s extension functions are used for adding functionality to existing classes without modifying their source code. This feature promotes cleaner code by keeping functionality modular and preventing class bloat. Extension functions are particularly useful in Android development when you need to add behavior to UI components or data models.
For example, you can create an extension function for the TextView class to simplify setting text:
Now, every TextView in your application can call setFormattedText() instead of manually formatting text in every usage. This approach makes the code cleaner and easier to maintain, especially in large Android projects with multiple UI components.
Another great use case is in handling data operations, where you can extend classes like List or Map with custom functions that make working with data more intuitive.
Lambda Expressions And Functional Programming
Kotlin supports lambda expressions, which are essentially anonymous functions that can be passed around as values. In Android development, lambdas can reduce the verbosity of your code, especially when working with collections or handling UI events.
For example, instead of writing a traditional loop to filter a list, you can use a lambda expression with Kotlin’s standard library functions like filter:
Lambdas are also heavily used in Android’s Jetpack libraries. For instance, in LiveData, you can use lambdas to observe changes in data:
Kotlin’s lambda expressions improve readability and reduce the amount of boilerplate code required for common tasks, making Android apps more efficient and maintainable.
Recursion And Tail-Recursive Functions In Kotlin
Recursion is a common programming technique where a function calls itself to solve smaller instances of a problem. In Android development, recursion is often useful for tasks like traversing hierarchical data structures (e.g., trees), handling file systems, or solving algorithmic problems. Kotlin fully supports recursion and offers additional optimizations, such as tail recursion, to improve performance.
A basic recursive function in Kotlin looks like this:
One common issue with recursion is the risk of a stack overflow when the recursion depth becomes too large. Kotlin addresses this with tail recursion, which optimizes recursive calls by treating them like loops, eliminating the risk of stack overflow.
In Kotlin, you can optimize a recursive function by adding the tailrec modifier, allowing the compiler to transform the recursive calls into an iterative process:
This approach is particularly useful when working with deep recursions, as it ensures that the function uses constant stack space, improving both performance and stability in Android applications.
Wrapping Up
Kotlin’s growth as a leading language in Android development is underpinned by its adaptability and support from Google, particularly with modern libraries like Jetpack Compose.
In addition to the core features covered, Kotlin also supports advanced design patterns and features like dependency injection, which can further streamline app architecture.
This makes Kotlin an essential tool for building scalable, maintainable, and efficient Android applications in today’s competitive mobile market.