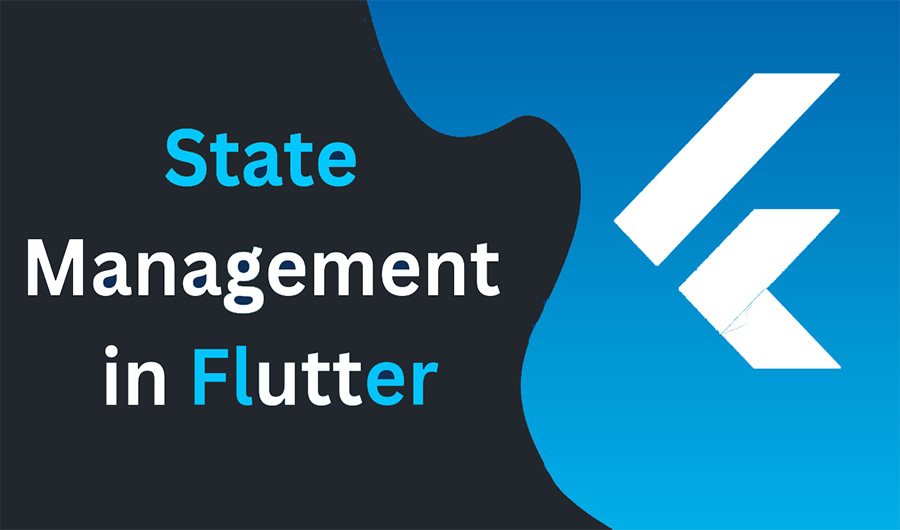
State Management in Flutter: Best Practices
Want to build a robust Flutter app? Then you can’t corner the state management aspect in the app development. Whether you are building an app for single or multiple platforms, state management is crucial as it improves separation of concern, simplifies code debugging, and provides better scalability.
Moreover, for the effective implementation of state management in Flutter apps, it’s important to hire Flutter developers with similar skill sets. Let’s delve into the best practices for implementing state management in the Flutter application along with the approaches and the use cases.
Understanding State in Flutter
Before diving into best practices, it is essential to understand what state is in the context of Flutter. State refers to any data that can change over time in an application. This includes user inputs, UI changes, data fetched from an API, or any variable that impacts the UI.
Types of State
Ephemeral State: This is the local state of a widget that is short-lived and often only relevant within the context of a single widget. For example, the state of a checkbox or a text field.
App State: This is the state shared across multiple parts of the application and can persist longer than ephemeral state. Examples include user authentication status, theme preferences, and data fetched from a remote server.
State Management Approaches in Flutter
Flutter provides multiple state management strategies, each with advantages and disadvantages. The following are the state management options that are most often used:
setState()
setState() is the most basic and direct way to manage state in Flutter. It is used to update the state of a StatefulWidget and trigger a rebuild of the widget tree.
Best Practices for Using setState()
- Keep State Localized: Use setState() for an ephemeral state that is relevant only within a single widget. This avoids unnecessary rebuilds of other parts of the widget tree.
- Avoid Complex Logic: Do not put complex business logic inside the setState() Instead, compute the new state separately and call setState() to apply the changes.
- Minimize State Changes: Only update the state that has changed. This minimizes performance overhead and ensures efficient rendering.
InheritedWidget and InheritedModel
InheritedWidget allows data to be passed down the widget tree and accessed efficiently by its descendants. InheritedModel is a more advanced version that allows finer-grained updates.
Best Practices for Using InheritedWidget and InheritedModel
- Use for App State: These are suitable for managing app state that needs to be accessed by multiple widgets throughout the app.
- Optimize Rebuilds: Use InheritedModel to optimize rebuilds by specifying which part of the state has changed, reducing unnecessary widget rebuilds.
Provider
Provider is a wrapper around InheritedWidget and is part of the official Flutter toolkit. It provides a more convenient and scalable way to manage state.
Best Practices for Using Provider
- Utilize ProxyProvider for Dependencies: Make sure that dependencies between providers are formed in the proper sequence by using ProxyProvider to handle them.
- Avoid Embracing Providers Too Much: A codebase that has too many providers might become complex and challenging to manage.
- Utilise ChangeNotifier and ValueNotifier: ChangeNotifier should be used for complex state changes involving numerous listeners, and ValueNotifier should be used for simple state changes involving just one listener.
Riverpod
Riverpod is a complete rewrite of Provider that improves upon its limitations. It offers a more robust and testable approach to state management.
Best Practices for Using Riverpod
- Use Scoped Providers: Scope providers to specific parts of the widget tree to avoid polluting the global state and making the app harder to maintain.
- Utilize AutoDispose: Use autoDispose to automatically clean up unused providers, reducing memory usage and potential leaks.
- Benefit from AsyncValue: Utilize AsyncValue to handle asynchronous state easily, providing loading, error, and data states in a single object.
BLoC/Cubit
BLoC (Business Logic Component) and Cubit are popular state management solutions for managing complex state with a clear separation of concerns between UI and business logic.
Best Practices for Using BLoC/Cubit
- Use Cubit for Simplicity: If your state management needs are straightforward, use Cubit, which is simpler and more lightweight than BLoC.
- Follow BLoC Pattern: When using BLoC, adhere to the BLoC pattern by separating events, states, and business logic to ensure clean architecture.
- Optimize Performance: Make sure that, by effectively utilizing the BLocBuilder widget, the BLoC or Cubit only rebuilds the portions of the user interface that require updating.
Redux
Redux is a state management tool that creates a single store representing the application state. The JavaScript Redux paradigm serves as its foundation.
Best Practices for Using Redux
- Keep Reducers Pure: Reducers should be pure functions without side effects to ensure predictable state changes.
- Leverage Middleware: Use middleware to handle side effects such as API calls, ensuring that the reducers remain pure.
- Normalize State: Normalize the state structure to avoid deep nesting and improve performance and maintainability.
General Best Practices for State Management
Regardless of the state management approach you choose, adhering to the following best practices will help maintain a clean and efficient codebase:
State Separation
- UI State vs. Business Logic: Separate UI-specific state from business logic to maintain a clean architecture. UI state should reside within widgets, while business logic should be handled by dedicated classes or services.
- Avoid Single Global State: Divide the state of your application into more manageable chunks. Give each component a state that is required, rather than utilizing a single, enormous pool of states for everything. This method makes your code more maintainable (easy to understand and modify) and modular (clearly separating concerns).
Testing
- Unit Tests for Logic: Write unit tests for your state management logic to ensure it behaves correctly in isolation. This is especially important for complex state transitions
- Widget Tests for UI: Use widget tests to verify that your UI responds correctly to state changes. This helps catch integration issues between the state and UI.
Performance Optimization
- Minimize Rebuilds: Use tools like Flutter’s DevTools to identify and minimize unnecessary widget rebuilds. This is crucial for maintaining a responsive UI.
- Use Memoization: Cache expensive computations and only recompute when necessary. This reduces the workload on the state management system and improves performance.
Consistency and Predictability
- Immutable State: Prefer immutable state objects to ensure state changes are predictable and easier to debug. This also helps prevent accidental state modifications.
- Clear State Transitions: Transitions between states should be well-defined and unambiguous. This enhances the code’s readability and maintainability.
Documentation and Naming Conventions
- Document State Changes: Document the purpose and expected behavior of different states and state transitions. This helps other developers (and your future self) understand the code.
- Consistent Naming: When naming state variables, methods, and classes, use standard conventions. This makes the code easier to read and update.
Conclusion
State management is an essential component of Flutter app development that has a big impact on your applications’ performance, maintainability, and dependability. The best state management solution for your project will depend on its complexity and particular needs, even if Flutter provides a wide range of options.
You may create reliable and manageable Flutter apps by adhering to the best practices described in this article, which include keeping state localized, isolating UI state from business logic, and performance optimization.
Whether you opt for the simplicity of setState(), the scalability of Provider, the robustness of Riverpod, the architectural clarity of BLoC/Cubit, or the centralization of Redux, understanding and applying these best practices will help you manage state effectively in your Flutter projects.