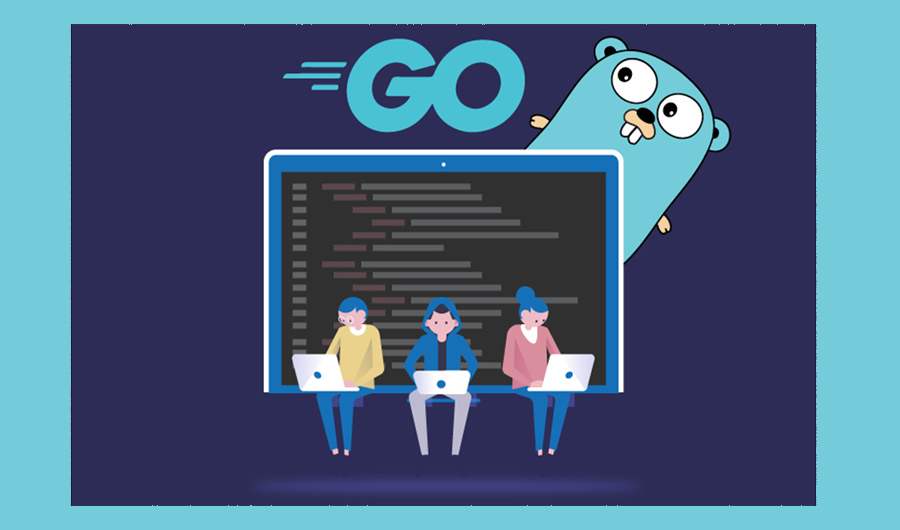
10 important Tips for Golang Developers
Golang is the modern-day web development language that eases the tasks of developers. It provides the opportunity for developmental professionals to add relevant features to web apps and relevant platforms. The language is based on C language and it is powered with static typing, concurrency management, and memory management.
Are you new to Golang web app development? Looking for smart tips to advance your Golang development projects swiftly? The programming codes should be written correctly so that the projects deliver optimal outcomes timely. Write the codes with proper understanding and also reduce the possibility of bugs in the Golang projects. This blog contains the right tips for Golang developer looking for some assistance in understanding coding tasks.
Let us get started….
Vital Tips For Golang Developers To Boost Development Projects
Concatenate Strings in Golang
The String concatenation means a combination of two strings in the formation of one string with the appending of the second string to the right of the first string to get optimal results. Looking for answers for how to efficiently concatenate strings in Go? The use of strings. Builder type is very crucial to concatenate strings efficiently in the Go platform.
Use of Single Responsibility Principle in Golang Programming
The idea of using the Single Responsibility Principle in Golang programming is meant to assign one responsibility for every class, function, or module in a program. It is the principle in REST API design to ensure specific endpoints have clear & relevant needs.
One responsibility is always necessary for each method or function. For functions with more than a single method or function, it can be hard to read & understand the use of the function. Also, testing & maintenance are harder due to it.
Let us check the bad and good ways to use the Single Responsibility Principle –
Writing Multiline Strings in Golang
The Go Standard Library has everything for Golang developers and for writing multiple strings in Golang, the use of built-in functionality delivers the best programming results. The strings in Golang are used in two main ways – Raw String Literals and Interpreted String Literals.
The backquote ` character in Raw String Literals will fit the Go functions in programming –
The expected outcome in this case will be –
Hi Bob
this
text has
multiline strings
Checking if a Map Contains a Key in Golang Programming
In Golang, developers can use the comma ok idiom for clearing the perspective related to checking a map containing a specific key in Golang.
If the key exists then it will be –
Avoid the Use of Global Variables in Programming
Often the developers look for the use of specific variables that can play a pivotal role in the outcomes. However, the Global variable makes the programming hard and thus the coding also becomes tough to test & maintain. It can be modified from different places as needed. In place of it, the developers should use local variables or pass variables as the relevant parameters for methods or functions in the language.
// bad
var count int
func incrementCount() {
count++
}
// good
func incrementCount(count int) int {
return count + 1
}
Use of Right Formatting In Go Programming
The right kind of formatting in the Golang functions will make the codes easy to read and understand. Using Golang’s built-in format will ensure proper use of code formatting.
// bad
func calculateAverage(nums []float64) float64 {
sum := 0.0
for _, num := range nums {
sum += num
}
return sum / float64(len(nums))
}
// good
func
calculateAverage(nums []float64) float64 {
sum := 0.0
for _, num := range
nums {
sum += num
}
return sum /
float64(len(nums))
}
Use of Functions and Method Short in Go
The proper use of methods and functions should be short & crisp. This ensures an easy understanding of codes. Also, the aim is to keep the functions & methods to lower than 50 lines of code and in cases the function or method is longer then break it into smaller parts as it will be more manageable.
// bad
func calculateAverageAndSum(nums []float64) (float64, float64) {
sum := 0.0
for _, num := range nums {
sum += num
}
average := sum / float64(len(nums))
return average, sum
}
// good
func calculateAverage(nums []float64) float64 {
sum := 0.0
for _, num := range nums {
sum += num
}
return sum / float64(len(nums))
}func calculateSum(nums []float64) float64 {
sum := 0.0
for _, num := range nums {
sum += num
}
return sum
}
Use Dynamic Arrays Like in Golang
Slice is referred to as the most desirable and convenient dynamic array and supports the Go methods or programs. Let us look at the top ways to perform some operations on Slice –
// Iterate over slice
for i, v := range s { // use range, inc order
// i – index
// v – value
}
for i := 0; i < len(s); i++ { // use index, inc order
// i – index
// s[i] – value
}
for i := len(s)-1; i >= 0; i– { // use index, reverse order
// i – index
// s[i] – value
}
// Function argument
func f(s []T) // s – passed by value, but memory the same
func f(s *[]T) // s – passed by refernce, but memory the same
// Append
a = append(a, b…)
// Clone
b = make([]T, len(a))
copy(b,a)
// Remove element, keep order
a = a[:i+copy(a[i:], a[i+1:])]
// or
a = append(a[:i], a[i+1:]…)
// Remove element, change order
a[i] = a[len(a)-1]
a = a[:len(a)-1]
Writing of Reliable Testable Codes
In Golang, writing codes are simple & flawless. The procedure is easy to identify and fix the bugs using reliable codes. Also, the use of test packages by Go ensures the writing of tests for simple coding.
// bad
func calculateAverage(nums []float64) float64 {
sum := 0.0
for _, num := range nums {
sum += num
}
return sum / float64(len(nums))
}
// good
func calculateAverage(nums []float64) float64 {
if len(nums) == 0 {
return 0.0
}
sum := 0.0
for _, num := range nums {
sum += num
}
return sum / float64(len(nums))
}func TestCalculateAverage(t *testing.T) {
nums := []float64{1.0, 2.0, 3.0, 4.0}
expected := 2.5
result := calculateAverage(nums)
if result != expected {
t.Errorf(“Expected %f but got %f”, expected, result)
}
}
Use of Variadic Functions
The right kind of method will ensure efficient programming in Golang. Variadic functions can be recalled easily using the number of trailing arguments like –
func variadicFunc(anotherArg int, args …string) ([]string, int) {
var arr []string
for _, s := range args {
arr = append(arr, s)
}
return arr, anotherArg
}
func main() {
fmt.Println(variadicFunc(129471294, “1”, “2”, “3”)) // [“1″,”2″,”3”]
}
Conclusion
Golang is leveraged by top brands across the world to address their website development tasks. Hope the tips mentioned above will help the simple writing of codes and ease out the programming output. Improve the overall Go programming needs with quality coding, and reduction of bugs, and also test the codes efficiently.